Multiple Inheritance
In C++, a class can inherit from more than one class. That is to say there can be two or more base classes for a derived class. This is called multiple inheritance.
Some other OOPS languages like Java, do not allow multiple inheritance.
Syntax for multiple inheritance is:
class cls-name:public base-cls1,public base-cls2,public base-cls3
{
/*code*/
}
The base class list is comma separated and has access specifier for each base class. Each of these classes must have been declared earlier.
Derived class objects contain sub-objects of each of the base classes.
We can give a simple example
class intern:public student,public employee{/*****/};
Let us look at another example.
In the above example, class Amphibian inherits from 2 classes - LAnimal and WAnimal. It has members of both these base classes. It can call both walk() and swim() functions. Size of a frog object = size of LAnimal+size of WAnimal.
The output of the program is
size of frog object is 8 size of rabbit object is 4 size of fish object is 4
walks
swims
Constructors
The derived class constructors and destructors in multiple inheritance will call all of the base class constructors and destructors. The order of constructor calls is same as declaration order of base classes in derived class definition.
Amphibian constructor in the earlier example, will call LAnimal constructor first, then WAnimal constructor and finally Amphibian constructor because our class header says
classs Amphibian:public LAnimal, public WAnimal
. And order of destructor calls is reverse of order of constructor calls.
output of program
B ctor A ctor Derived ctor
Here MI class declaration uses B followed by A in base class specification. So B ctor is called before A ctor when MI object is created even though in member initialization list, A ctor is called first.
Dreaded Diamond problem
When there are multilevel and multiple inheritances together, derived class may contain more than one instance of an ancestor class. In such a situation, calling a method from this ancestor class throws an ambiguity error.
When we try to compile this program, there will be syntax errors as shown.
g++ drdia.cpp
drdia.cpp: In function ‘int main()’:
drdia.cpp:28:12: error: request for member ‘getm’ is ambiguous
cout<<obj.getm();/*error*/
^
drdia.cpp:8:6: note: candidates are: int A::getm()
int getm(){return m;}
^
drdia.cpp:8:6: note: int A::getm()
drdia.cpp:29:6: error: request for member ‘print’ is ambiguous
obj.print();/*error*/
^
drdia.cpp:9:7: note: candidates are: void A::print()
void print(){cout<<"m"<<m<<endl;}
^
drdia.cpp:9:7: note: void A::print()
The reason of these errors is obj has members of A class duplicated.
When calling print() or getm() , compiler is unable to decide whether to call these functions of B class or of C class.
This is the dreaded diamond problem because of the shape of inheritance diagram.
To avoid this error, virtual inheritance is used.
Virtual Inheritance
Virtual inheritance is the mechanism by which only one copy of base class are inherited by grand child derived classes. Let us look at the a diagram.
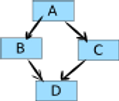
If virtual inheritance is not used in the above diagram, if B and C classes are inherited from class A, and D is inherited from both B and C, then D class will 2 copies of members of A - one from B class and another from C class.
This can be avoided by using virtual inheritance. Virtual inheritance is achieved by using keyword virtual with the base class name.
Both B and C classes use virtual inheritance. So D which inherits from both B and C, it is inheriting only one sub-object of A class. So there is only one data member called m in D class and only one print() function in it. There will not be any ambiguity when obj.print() function is called.
Also observe that obj causes A class constructor to be called only once and A destructor to be called only once.