In this page we will see function overloading and default parameters to functions. Function overloading is defining multiple functions with same name.
Default parameters to functions are parameters are the values which are taken for parameters if one is not supplied.
Function Overloading
To understand the need for function overloading, let us look at what happens in procedure-oriented language like C . Let us say you want to write a function add to add two integers.
int add(int a,int b)
{
return a+b;
}
But will this function be able to add two floats and return the answer? No, it will not.
We can call the function with float arguments. But the function converts them to integers implicitly and returns an integer answer.
So, we must write another function for float parameters which is very much similar to the previous function except for data types.
float add(float a,float b)
{
return a+b;
}
Wait, wait. But, this is wrong. We can't have two functions with the same name doing the same task. Program will not compile.
So we have to change the name of second function to addf()
float addf(float a,float b)
{
return a+b;
}
Similarly we have to write addd() for double values, addar() for arrays and so on.
We are writing so many functions with confusing names. But they all do same operations and should be able to have same name.
C++ solves this problem by letting you have overloaded functions - functions with same name but different number/type of parameters.
Functions having same name but different number and/or type of parameters are called overloaded functions.
When an overloaded function is called, depending on the type and number of arguments, the correct function is invoked by the compiler.
The previous two functions - add() and addf() , we have seen above can both be called add() in C++.
Let us see this concept with a complete program.
In the example above, we have written two function print(int num) and print(float num). Both have same name but one has an integer parameter and the other has a float parameter.
When print() is called with a, the first function print(int num) is called because a is an integer. And print(b) will call print(float num).
Output of the program is
The integer is 22
The floating point number is 1.5
What is the commented line in the program? What happens if we uncomment that statement?
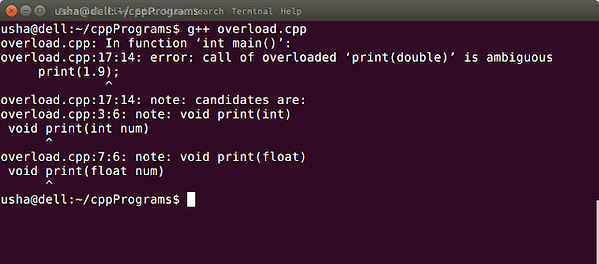
Now when we compile the program, we get a syntax error - ambiguity error.
What went wrong? In C++, the arguments are implicitly converted to type of parameters during a function call . e.g. if there is a function int square(int) and it is called as square(1.4), the number 1.4 is converted to an integer (1) and function is called.
But here in this example, we have two functions with different types of parameters int and float. So there is a confusion - Should the number 1.9 be converted to int or float?
But, wait! Isn't 1.9 already a float literal?
No. It is not.
1.9 is a double. Unqualified real literals are double (precision)s in C++. A suffix of f or F, will make the literal as float number.
We can correct the error by saying print(1.9f).
Or we can use explicit conversion.
Now we have converted 1.9 to float using explicit conversion. And now there will be no error in the program.
The output is
The integer is 22
The floating point number is 1.5
The floating point number is 1.9
Default values for parameters
Functions can specify default values for one or more parameters. If an argument is not passed for that parameter, then its default value will be taken.
Let us look at an example.
Here sum function takes 4 parameters. But if you omit d, then it is taken as 0.
So the following function call is valid
int s1 = sum(m,n,p);//d is 0
Similarly if we omit 3rd argument, the function call is still valid and c is taken as 0.
Let us call the function with different possibilities.
int s1 = sum(10,11,3,4);//s1 is 28
int s2 = sum(12,13,14);//s2 is 39
int s3 = sum(15,16);//s3 is 31
int s4 = sum(17);//error. no default value for b
The last call to sum function with just one argument is wrong. Because the function has default values for c and d, not for b.
Where to specify values?
We can ideally specify default values in declaration of function.
Let us look at another example.
#include<iostream>
using namespace std;
int product(int n1,int n2=1,int n3=1);
int main()
{
cout<<"Product of 2 numbers is "<<product(10,20);
cout<<endl;
cout<<"Produc of 3 numbers is "<<product(10,20,30);
cout<<endl;
cout<<"Productc of one number is "<<product(31)<<endl;
return 0;
}
int product(int n1,int n2 ,int n3 )
{
return n1*n2*n3;
}
In the example above, we have defined product() function with default parameters. Which means this function can be called without these two parameters.
Next let us look at another program with default parameters here.
But this program does not compile.
Why?
The compilation error says
error: default argument missing for parameter 2 of ‘int product(int, int, int)’
As we gave default value to a, but not b, we get an error.
This means that we start by giving default value to rightmost parameter and then proceed towards left.
We can not omit a parameter without value in the middle.
Exercises:
1) Write a program to overload product function - one with 3 integer parameters, another with 3 double parameters and third with 2 integer and one float parameter.
2) Write a program to overload average function - to find average of 2 integers, 2 floats and 3 integers.
3) Write a function to convert a number to given base (e.g. to binary, octal or hexadecimal numbers). Function must take number and base as parameters. If base is not provided, it should be taken as 8 - octal.
4) Write a function to read the elements of an array. The function must take array and its size as parameters. If size is not specified, it must be taken as 5.